Javascript Timer Example
The aim of this example is to explain the timing events in javascript.
The javascript window.setTimeout(function, milliseconds)
function is used to execute a function, after waiting a specified number of milliseconds. This method returns a timeout id.
Notice,
setTimeout
and window.setTimeout
are essentially the same, the only difference being that in the second statement we are referencing the setTimeout
method as a property of the global window object.The javascript clearTimeout(timeoutID)
function is used to stop the given timer.
Following tips are very important when working with this function
- The function is only executed once. If you need to repeat execution, use the
setInterval()
method. - Use the clearTimeout() method to prevent the function from running.
1. HTML
First of all you need to create a simple html documents.
timerExample.html
<!DOCTYPE html> <html> <head> <title>javascript timer example</title> </head> <body> </body> </html>
2. Javascript Timer Examples
2.1 Show alert after 3 seconds
This example sets a timer to call the given method after 3 seconds. As you can notice, the setTimeout(function, milliseconds)
function takes two parameters, the first is the method name and the second is the interval time in milliseconds.
Click a button. Wait 3 seconds, and the page will alert “Hello”:
<button onclick="setTimeout(myFunction, 3000)">Try it</button> <script> function myFunction() { alert('Hello'); } </script>
The result in the browser would be:
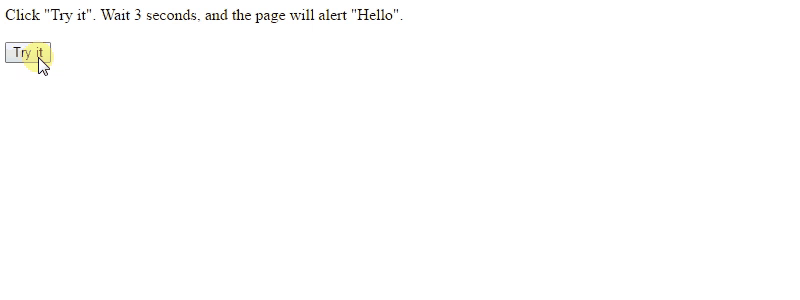
2.2 Stop javascript timer
The javascript clearTimeout(timeoutID)
is used to stop the timer by using the given timeoutID. The javascript setTimeout(function, milliseconds)
function returns a timeout id that can be passed to this method.
<button onclick="myVar = setTimeout(myFunction, 2000)">Try it</button> <button onclick="clearTimeout(myVar)">Stop it</button> <script> function myFunction() { alert('Hello'); } </script>
The result in the browser would be:
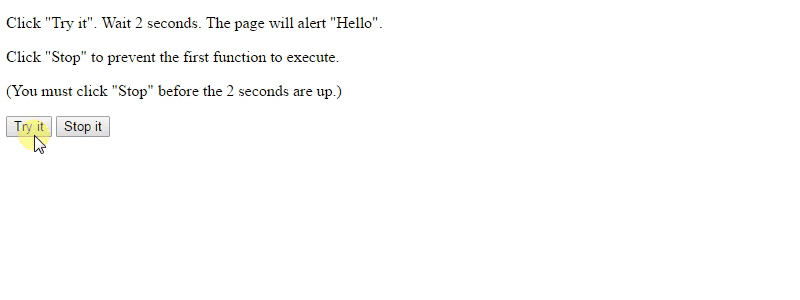
2.3 O’clock example
As you can notice, in the line 4 of the following javascript code, we call the startTime()
method to show o’clock and set a timer. This timer will call the startTime()
method after a half of second. That will help us to update the o’clock values.
<div id="txt"></div> <script> startTime(); function startTime() { var today = new Date(); var h = today.getHours(); var m = today.getMinutes(); var s = today.getSeconds(); // add a zero in front of numbers<10 m = checkTime(m); s = checkTime(s); document.getElementById("txt").innerHTML = h + ":" + m + ":" + s; var t = setTimeout(function(){ startTime() }, 500); } function checkTime(i) { if (i < 10) { i = "0" + i; } return i; } </script>
The result in the browser would be:
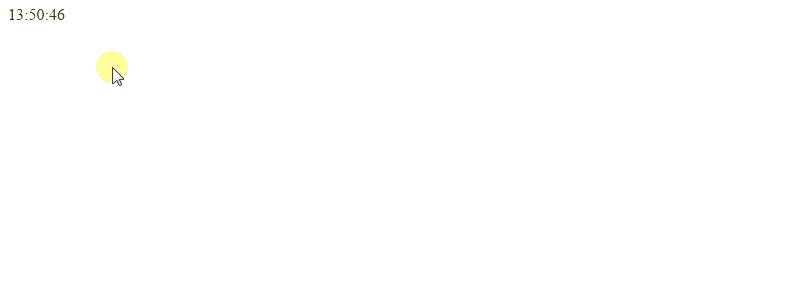