Angular.js Routing Example
Hey geeks! Today’s example is related with routing in AngularJS. What I firstly found out, while searching over the net for similar resources, is that there isn’t yet any simple example, suitable for an Angular newbie. So, I’ll try to keep today’s post as simple as I can.
1. What is routing in a web app
Generally, web applications make use of readable URLs that describe the content that resides there. A common example could be clicking on a homepage’s link: this means that a back-end action is being executed, that results to a different view on the client-side. We often confirm similar situations after interacting in the root of a web app ( /
or index.html
), by noticing a change to the browser’s url bar.
2. Today’s example’s concept
In this example we will demonstrate a simple page navigation application. Suppose a homepage with two links and each one of them will redirect to a specified page.
To get a better understanding of our concept, we ‘ll here implement an inline navigation. This means that we want our pages’ content to be displayed inside the initial/home page.
AngularJS provides the ngView
directive to implement the fore-mentioned functionality. Specifically, ngView
directive complements the $route service by including the rendered template of the current route into the main layout file. That is, each time the current route changes, the included view changes with it according to the configuration of the $route
service.
So, keeping in mind that our index.html
contains a simple sentence with two links provided, assume we want to display the rendered templates (according to the clicked links) below that sentence; this should seem like:
<p>Jump to the <a href="#first">first</a> or <a href="#second">second page</a></p> <div ng-view> </div>
As you see, the anchors’ targets are already named, so what is left, is to configure the respective routings for Angular.
So, at this point we should have a complete homepage:
index.html
<!DOCTYPE html> <html> <head> <link rel="stylesheet" href="https://www.webcodegeeks.com/wp-content/litespeed/localres/aHR0cHM6Ly9tYXhjZG4uYm9vdHN0cmFwY2RuLmNvbS8=bootstrap/3.3.2/css/bootstrap.min.css"> <script src="http://ajax.googleapis.com/ajax/libs/angularjs/1.2.26/angular.min.js"></script> <script src="http://ajax.googleapis.com/ajax/libs/angularjs/1.2.26/angular-route.min.js"></script> <script src="script.js"></script> </head> <body ng-app="RoutingApp"> <h2>AngularJS Routing Example</h2> <p>Jump to the <a href="#first">first</a> or <a href="#second">second page</a></p> <div ng-view> </div> </body> </html>
In AngularJS, we can use the ngRoute
module for routing and deeplinking services and directives.
Here are some important points before going on:
- In order to use the
ngRoute
module, you have to includeangular-route.js
to your app, which, obviously, has to be loaded, after including theangular.js
script. - The routings we need, have to be configured inside the module’s functionality, so it would be easier to define our module in a separate file,
script.js
. - You have to provide same name for the
ng-app
tag (in html file that contains the Angular app) and the module’s definition.
2.1 Defining the module
Now, let’s define the Angular module, by providing our app’s name (as in index.html
) and declaring that it depends on the ngRoute
module; the last words mean that we have to “inject” ngRoute
into our module ( script.js
), like this:
angular.module('RoutingApp', ['ngRoute']);
That’s why we had to include the angular-route.js
file in our app.
In order to use ngRoute
, we have to call the angular.config
method:
angular.module('RoutingApp', ['ngRoute']) .config(function() { });
As you noticed, I also created an anonymous function inside the method, ’cause, otherwise, we ‘ll get a script error from our browser, as angular.config
method requires calling it with a function.
From the official documentation, we can use the $routeProvider
to configure Angular’s routes, so we should pass $routeProvider
as a parameter in our anonymous function:
angular.module('RoutingApp', ['ngRoute']) .config( ['$routeProvider', function($routeProvider) { }]);
The $routeProvider
‘s method to add a new route definition to the $route
service is when(path, route)
:
path
corresponds to the requested from the client path.route
is an object parameter and contains mapping information that have to be assigned while matching the requested route (i.e. we may want to handle the newly registered route with a specific controller;controller
property is responsible for this scope).
You can read about the rest of route
‘s object properties, but as I fore-mentioned, here, we ‘ll implement a simple routing between two html files, so, I’ll only use the templateUrl
.
Please take a look at the module’s final structure:
script.js
angular.module('RoutingApp', ['ngRoute']) .config( ['$routeProvider', function($routeProvider) { $routeProvider .when('/first', { templateUrl: 'first.html' }) .when('/second', { templateUrl: 'second.html' }) .otherwise({ redirectTo: '/' }); }]);
Now, let me explain: the first when
means that when the /first
is requested as a route, the first.html
will be loaded. Same for the “second”.
The $routeProvider
‘s otherwise(params)
method sets route definition that will be used on route change when no other route definition is matched. Practically, this means, that if the client requests a route that isn;t defined in the when
method, this method will be executed. Imagine this as a general if-else statement.
In our case, I assume we want to display just the homepage ('/'
), when no route definition gets matched.
3. Demo
Firstly, please take a look at this post, just to understand why you should deploy this app in a local server rather than just executing it in a browser.
Access the web app from your local server:
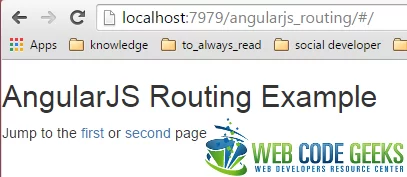
Now, click on “first”:
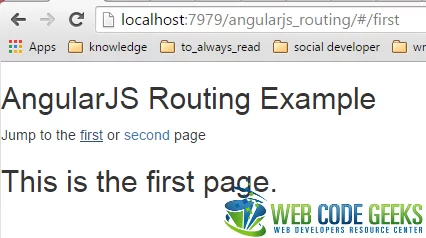
Same, for the “second”:
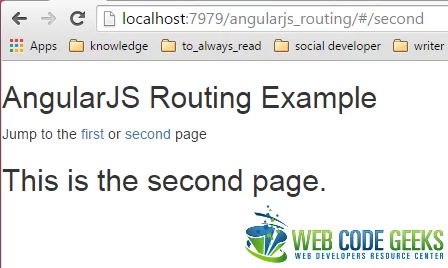
4. Download the project
This was an example of AngularJS Routing.
You can download the full source code of this example here : angularjs_routing.zip
Gracias.
Quito.-Ecuador
“La Mitad del Mundo”
Hi Miguel, it’s nice to hear that it helped you :) .
I need your help when i call jason file through $http function it dnt print the data of jason file by using route function here is the function
Script file:
var app = angular.module(‘myApp’, []);
app.config([‘$routeProvider’,
function($routeProvider) {
$routeProvider.
when(‘/’, {
templateUrl: ‘category.html’,
controller: ‘myCtrl’
}).
when(‘/route2′, {
templateUrl: ’empty.html’
}).
otherwise({
redirectTo: ‘/’
});
}]);
app.controller(‘myCtrl’, function($scope, $http) {
$http.get(“http://api.remix.bestbuy.com/v1/categories?apiKey=uh8w84g28ckp7m6swzpx5vkc&format=json”)
.success(function (response) {$scope.sample =response.categories;});
});
Html file:
route which i gave :
Products
{{product.name}}
if you can solve this please mail me on my email id
Thanks it is good article really. Get more with other example with source code at http://angularjsaz.blogspot.com/2015/10/angularjs-routing-aspnet-mvc-example.html
Nice, really good a simple example what I need. Can this upgrade to v1.4.7?
Examples are the best way to learn AngularJS. Assume you already know JAVASCRIPT, then what more you required? Only Examples are good enough to get basics of AngularJS. Look at this link http://jharaphula.com/category/programming-solutions/learn-angularjs-with-examples here I collected all the fundamentals of AngularJS. its easy n fun to learn.
Very interesting post, this is my first time visit here. I found so many interesting stuff in your blog especially its discussion..thanks for the post!