Javascript Date Validation Example
The aim of this article is to validate date input in javascript. When getting information from a user for insertion into a database, or use in other processing, it’s important to control what the user can enter. Otherwise you can end up with values in the database that have no relation to reality.
Javascript does not provide any method to achieve validation of date or even quite a lot of other kinds of validation. So, it makes sense the the developer should take the task of creating a function which will handle validation of date in aspects of format, number limits etc.
Through this article, you will be shown easy functional ways to make this validation. Remember that your code shall be as clean as possible.
1. Document Setup
To begin, create a new HTML document and add the very basic syntax for writing HTML and Javascript like so:
js-date-validation.html
<!DOCTYPE html> <html> <head> <title>Javascript Date Validation Example</title> </head> <body> <!-- HTML SECTION --> <!-- JAVASCRIPT SECTION --> <script type="text/javascript"> </script> </body> </html>
Next, we’ll be adding the elements we need inside a form
since this is going to be ‘submitted’.
<!-- HTML SECTION --> <form method="POST" onsubmit="return checkDate(this);"> <span>Please enter a date below:</span><br/> <input type="text" name="date" placeholder="dd/mm/yyyy"> <br/><input type="submit" value="Validate"> </form>
The onsubmit="return checkDate(this);
will serve as a way to trigger the function of validation as soon as the form is submitted. This function will be implemented below. For now, we’d have this view:

2. Implementing a Date Validation Function
The next step is validating the form’s only input, so that it matches that of a date.
2.1 Example 1
In this first example, we’re giving an easy to understand dd/mm/yyyy
date validation.
<!-- JAVASCRIPT SECTION --> <script type="text/javascript"> // new function to handle validation function checkDate(form) { // regular expression to match required date format re = /^(\d{1,2})\/(\d{1,2})\/(\d{4})$/; // ^ means begin, \d means expect a digit within {n1, n2}, $ means end with. if(form.date.value != '') { // check to see the input is not left empty if(regs = form.date.value.match(re)) { // check and save the general format matching // day value between 1 and 31 if(regs[1] < 1 || regs[1] > 31) { // regs[1] means the first value given alert("Invalid value for day: " + regs[1]); // alert user to check day again form.date.focus(); // give focus to the input field return false; } // month value between 1 and 12 if(regs[2] < 1 || regs[2] > 12) { alert("Invalid value for month: " + regs[2]); form.date.focus(); return false; } // year value between 1 and 2016 if(regs[3] < 0 || regs[3] > new Date().getFullYear()) { alert("Invalid value for year: " + regs[3] + " - must be between 1 and " + (new Date()).getFullYear()); form.date.focus(); return false; } } else if(isNaN(form.date.value)) { // check if input is not a number alert("You entered a string."); form.date.focus(); return false; } } else { alert("No input was given!"); form.date.focus(); return false; } } </script>
Let’s now try 5 different inputs:
1. Give an invalid date:
2. Give an invalid month:
3. Give an invalid year:
If more that one of the requirements is not met, like both date and month is invalid, only the alert of the first invalid input argument will be shown and you will be focused to the input again.
4. Do not write anything (empty input):
5. Write a string, not a number:
2.2 Example 2
This is basically the same, but this time, we check the number limit for day, month and year inside the regular expression:
<!-- JAVASCRIPT SECTION --> <script type="text/javascript"> function checkDate(form){ // control the number range inside the regular pattern var datePattern = /^[0-3]?[0-9]\/[0-1]?[0-9]\/[0-2][0-9][0-9][0-9]$/; if(form.date.value.match(datePattern)) { alert("Correct Date"); } else alert("Invalid Date"); } <script>
This will throw the message “Invalid Date” for any input not matching the one in the pattern. So we’re not checking each number individually, but using the regular expression alert the user if the date is correct or not. Below is shown a out of bounds date.
2.3 Example 3
Lastly, let’s also say something about different date formats. For example, the U.S. uses this format mm/dd/yyyy
. Changing our code to match this kind of format would result in the same one as before except the regular expression line:
var datePattern = /^[0-1]?[0-9]\/[0-3]?[0-9]\/[0-2][0-9][0-9][0-9]$/;
Now we can enter a date in the mm/dd/yyyy
format like so:
In a similar manner, other date formatting like dd.mm.yyyy
could be achieved just by changing the regular expression:
var datePattern = /^[0-3]?[0-9]\.[0-1]?[0-9]\.[0-2][0-9][0-9][0-9]$/;
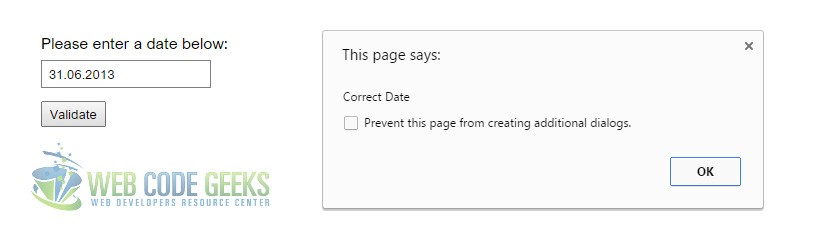
3. The Final Example
The final example will also handle something we haven’t mentioned: the days of a month that vary from year to year, like february. For this we add another function which will return the date of a specific month of a year and then we’ll use this function to compare the maximum day of that month with the given value.
<!-- JAVASCRIPT SECTION --> <script type="text/javascript"> function daysInMonth(month,year) { return new Date(year, month, 0).getDate(); } //new function to handle validation function checkDate(form) { // regular expression to match required date format re = /^(\d{1,2})\/(\d{1,2})\/(\d{4})$/; // ^ means begin, \d means expect a digit within {n1, n2}, $ means end with. if(form.date.value != '') { // check to see the input is not left empty if(regs = form.date.value.match(re)) { // check and save the general format matching // day value between 1 and 31 if(regs[1] < 1 || regs[1] > 31 || regs[1] > daysInMonth(regs[2], regs[3])) { // check for month days alert("Invalid value for day: " + regs[1]); // alert user to check day again form.date.focus(); // give focus to the input field return false; } // month value between 1 and 12 if(regs[2] < 1 || regs[2] > 12) { alert("Invalid value for month: " + regs[2]); form.date.focus(); return false; } // year value between 1 and 2016 if(regs[3] < 0 || regs[3] > new Date().getFullYear()) { alert("Invalid value for year: " + regs[3] + " - must be between 1 and " + (new Date()).getFullYear()); form.date.focus(); return false; } } else if(isNaN(form.date.value)) { // check if input is not a number alert("You entered a string."); form.date.focus(); return false; } } else { alert("No input was given!"); form.date.focus(); return false; } } <script>
Now everything is checked:
4. Conclusion
To conclude, it is important to keep it simple. Sometimes, regular expressions might confuse you, but that is a really short way of handling it. However, feel free to be more specific in your code using the last example for your own clarity. Of course, you can also think of other ways of validating dates in javascript, but this is one functional example ready to be used.
5. Download the source code
You can download the full source code of this example here: Javascript Date Validation