Populate Oracle JET table from JSON based REST Web Service
Before starting with this post first go through my previous post about using JET with JDeveloper. Here I am using the same JET nav drawer template application. In this post, we’ll see how to consume JSON based REST Web Service and populate data in Oracle JET table (oj-table) component
We have URL of REST Web Service – http://dummy.restapiexample.com/api/v1/employees, it returns a list of employees and their details.
Let’s see the implementation part
Add an oj-table component in the dashboard.html page and define the required number of columns, this web service returns multiple details of an Employee so I have taken 4 columns for Id, Name, Salary, and Age
<div class="oj-hybrid-padding"> <h1>Dashboard Content Area</h1> <div id="div1"> <oj-table id='table' aria-label='Departments Table' data='[[datasource]]' selection-mode='{"row": "multiple", "column": "multiple"}' dnd='{"reorder": {"columns": "enabled"}}' scroll-policy='loadMoreOnScroll' scroll-policy-options='{"fetchSize": 10}' columns='[{"headerText": "ID", "field": "emp_id", "resizable": "enabled"}, {"headerText": "Name", "field": "emp_name", "resizable": "enabled"}, {"headerText": "Salary", "field": "emp_sal", "resizable": "enabled"}, {"headerText": "Age", "field": "emp_age", "resizable": "enabled"} ]' style='width: 100%; height: 400px;'> </oj-table> </div> </div>
Now see the code of dashboard.js, Read comments to understand the javascript code, Here getJSON() method of jQuery is used to call REST Web Service as jQuery is a part of Oracle JET libraries.
/* * Your dashboard ViewModel code goes here */ define(['ojs/ojcore', 'knockout', 'jquery', 'ojs/ojinputtext', 'ojs/ojtable', 'ojs/ojarraytabledatasource'], function (oj, ko, $) { function DashboardViewModel() { var self = this; //Define a Knockout observable array and name it tabData self.tabData = ko.observableArray(); //Use jQuery method to call REST Web Service $.getJSON("http://dummy.restapiexample.com/api/v1/employees").then(function (employees) { $.each(employees, function () { //Push data in Observable array self.tabData.push( { emp_id : this.id, emp_name : this.employee_name, emp_sal : this.employee_salary, emp_age : this.employee_age }); }); }); //Pass observable array in utility class to show data in table self.datasource = new oj.ArrayTableDataSource(self.tabData, { idAttribute : 'emp_id' }); . . .
tabData is a knockout observable array that is defined to hold returned value and you can see that self.tabData.push is used to push web service data into this array and at last a JET utility class ArrayTableDataSource is used to convert this observable array in tabular format data.
Here id, employee_name, employee_salary, employee_age are the attribute’s name returned by Web Service in JSON format.
Now right click on the index.html file in JDeveloper and choose run
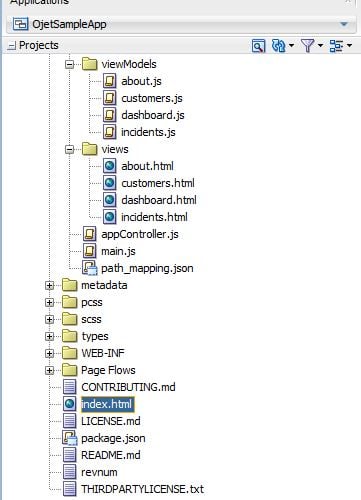
and see Oracle JET table in the browser, Data is populated from Web Service. In the same way, you can try some other Web Service URLs.
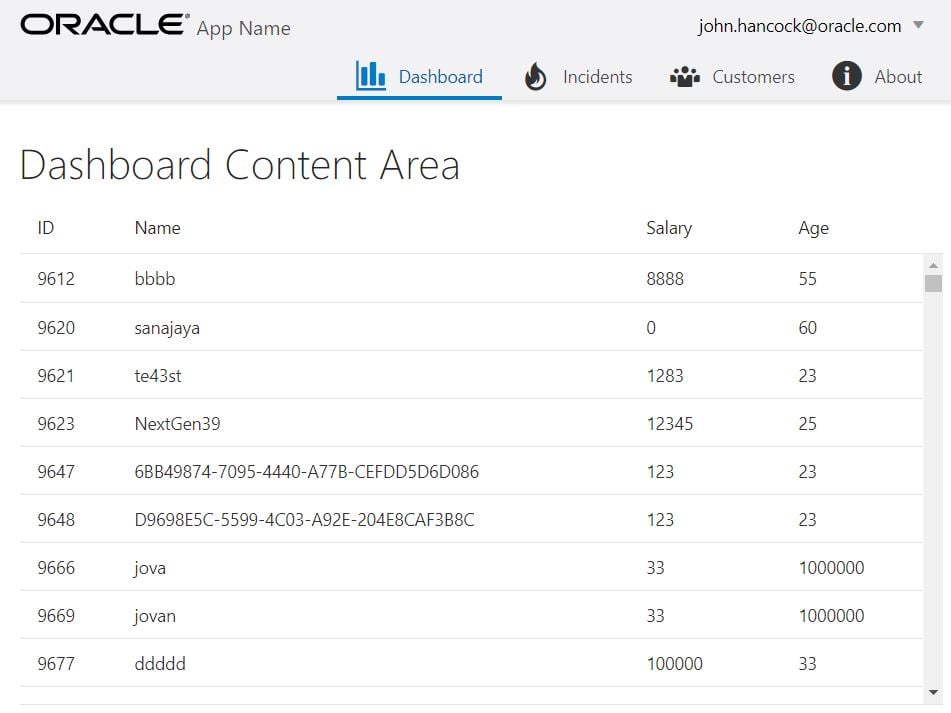
All Done
Cheers
Happy Learning
Published on Web Code Geeks with permission by Ashish Awasthi, partner at our WCG program. See the original article here: Populate Oracle JET table from JSON based REST Web Service Opinions expressed by Web Code Geeks contributors are their own. |